Cranfield University is interfacing data and systems that weren’t originally intended to work together seamlessly. That usually means loading data from legacy, or open source formats into proprietary systems, or vice versa. The challenge, on this occasion, was taking a GeoJSON feed of points and layering them onto a web map built with the ArcGIS JavaScript API. Some open source web mapping platforms, such as Leaflet, offer a simple library function for importing a GeoJSON feed as a vector layer for your map. No such luck in this case. The solution is reasonably simple and essentially involves building a JSON object to look something like an ArcGIS FeatureService. In fact the FeautureLayer contructor can take a JSON object as a parameter instead of an online FeatureService, we just need to be aware of the exact data format that it’s expecting and programatically build that object up from the GeoJSON (or whatever other data) that we have. First we create an empty feature collection:
var featureCollection = { "layerDefinition": null, "featureSet": { "features": [], "geometryType": "esriGeometryPoint" } };Then give the feature collection a layer definition:
featureCollection.layerDefinition = { "geometryType": "esriGeometryPoint", "objectIdField": "ObjectID", "drawingInfo": { "renderer": { "type": "simple", "symbol": { "type" : "esriSMS", "style" : "esriSMSCircle", "color" : [ 67, 100, 255, 70 ], "size" : 7 } } }, "fields": [{ "name": "ObjectID", "alias": "ObjectID", "type": "esriFieldTypeOID" }, { "name": "some_other_field", "alias": "some_other_field", "type": "esriFieldTypeString" } ] };At this point our
featureCollection
object is in the correct format to be passed in to a FeatureLayer:
featureLayer = new FeatureLayer(featureCollection, { id: 'myFeatureLayer', mode: FeatureLayer.MODE_SNAPSHOT });The Feature can now be added to the map. Note that the layer doesn’t actually contain any data at this stage, so wait for the layer to finish being added then begin the task of loading in the external data and adding points to our FeatureLayer. The lat/long coordinates of each point, i, that we’re after are stored within the GeoJSON object as
response.features[i].geometry.coordinates[0]
and response.features[i].geometry.coordinates[1]
function requestData() { var requestHandle = esriRequest({ url: "data/sample.json", callbackParamName: "jsoncallback" }); requestHandle.then(requestSucceeded, requestFailed); } function requestSucceeded(response, io) { //loop through the items and add to the feature layer var features = []; array.forEach(response.features, function(item) { var attr = {}; //pull in any additional attributes if required attr["some_other_field"] = item.properties.Here is the resulting map: [caption id="attachment_471" align="aligncenter" width="338"]; var geometry = new Point(item.geometry.coordinates[0], item.geometry.coordinates[1]); var graphic = new Graphic(geometry); graphic.setAttributes(attr); features.push(graphic); }); featureLayer.applyEdits(features, null, null); } function requestFailed(error) { console.log('failed'); }
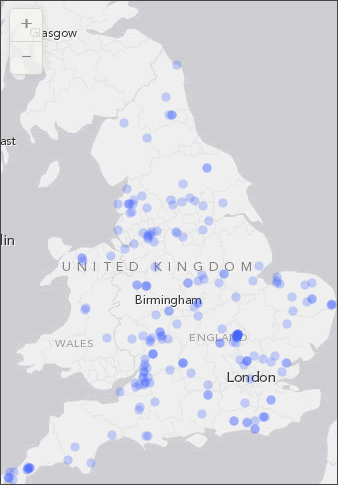
"esri/map",
"esri/layers/FeatureLayer",
"esri/request",
"esri/geometry/Point",
"esri/graphic",
"dojo/on",
"dojo/_base/array",
"dojo/domReady!"
]]>